Working with Files in Python: A Comprehensive Guide
Python provides a variety of powerful tools and functions for handling files. Whether you need to read data from a file, write data to a file, or handle exceptions during file operations, Python makes these tasks straightforward and efficient. In this guide, we will explore the essential concepts of file input/output operations in Python, including reading, writing, handling exceptions, and working with file objects.
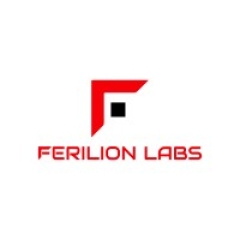