Unleashing the Power of Control Flow: Exploring Conditional Statements, Loops, Functions, and Modules in Python
Python, being a versatile programming language, provides several control flow mechanisms that allow developers to create complex and dynamic programs. In this article, we will explore conditional statements, loops, and other control flow mechanisms in Python programming.
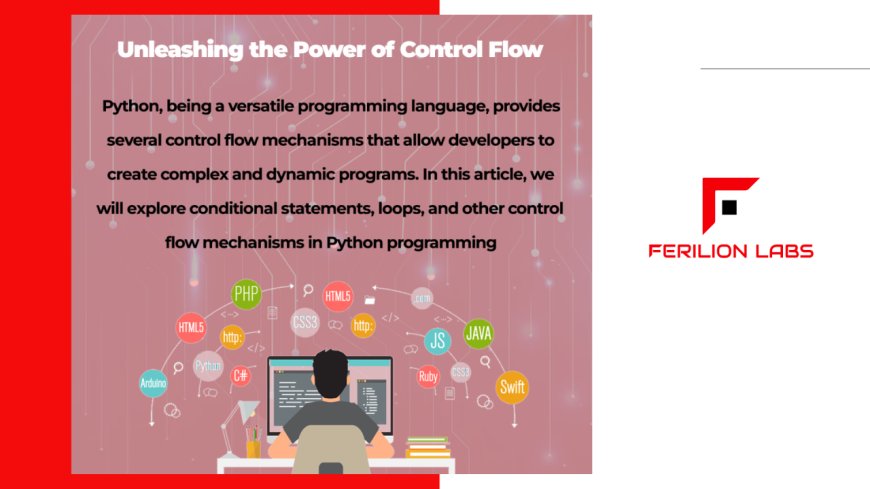
Python, being a versatile programming language, provides several control flow mechanisms that allow developers to create complex and dynamic programs. In this article, we will explore conditional statements, loops, and other control flow mechanisms in Python programming.
Conditional Statements: If, Elif, or Else
Conditional statements are used to execute different blocks of code based on certain conditions. In Python, we have three types of conditional statements: if, Elif, and else.
The "if" statement is the most basic type of conditional statement. It allows us to execute a certain block of code only if a specified condition is true. For example:
if condition:
# code block executed if the condition is true
'''
The 'elif' statement is used to check for additional conditions if the previous conditions are not met. It stands for "else if". Multiple 'elif' statements can be chained together to check for multiple conditions. For example:
if condition1:
# code block executed if condition 1 is true
elif condition2:
# code block executed if condition2 is true
else:
# code block executed if none of the conditions above are true
'''
Loops: For and While Loops
Loops are used to repeat a certain block of code multiple times until a specified condition is met. In Python, we have two types of loops: for and while loops.
The "for" loop is used to iterate over a sequence (such as a list, tuple, or string) or other iterable objects. It allows us to perform a set of operations on each item in the sequence. For example:
For items in sequence:
#code block executed for each item in the sequence
'''
The "while" loop is used to repeatedly execute a block of code as long as a certain condition is true. It is useful when we do not know the number of iterations in advance. For example:
while condition:
# code block executed as long as the condition is true
'''
Control Flow Mechanisms
Python provides additional control flow mechanisms to modify the flow of execution within loops and conditional statements.
The "break" statement is used to exit the current loop prematurely. When encountered, it immediately terminates the loop and resumes execution at the next statement after the loop. This can be useful when we want to stop the loop based on a certain condition.
The "continue" statement is used to skip the rest of the current iteration of a loop and move to the next iteration. It allows us to skip certain iterations based on a certain condition, without exiting the entire loop.
Functions and Modules in Python
Functions and modules are powerful tools in Python for organizing and reusing code.
Functions are blocks of reusable code that perform a specific task. They allow us to write modular and reusable code, improving code readability and maintainability. Functions are defined using the "def" keyword, followed by the function name and any required parameters. For example:
'''python
def function_name(parameter1, parameter2):
#code block defining the function's behavior
# perform operations using the parameters
'''
Modules, on the other hand, are files containing Python code that can be imported and used in other programs. They enable code organization by grouping related functions and classes. We can create our modules or make use of built-in modules provided by Python. Modules are imported using the 'import' keyword. For example:
'''python
import module_name
# Access functions and variables from the module
module_name.function_name()
'''
We have explored control flow mechanisms in Python, including conditional statements (if, elif, else), loops (for and while loops), as well as functions and modules. These concepts are fundamental to writing efficient and structured code in Python. By understanding and applying these control flow mechanisms, you can create dynamic and reusable programs with ease.
What's Your Reaction?

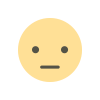
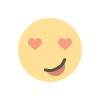



