10 Essential Tips for Developing GUIs with TKinter in Python
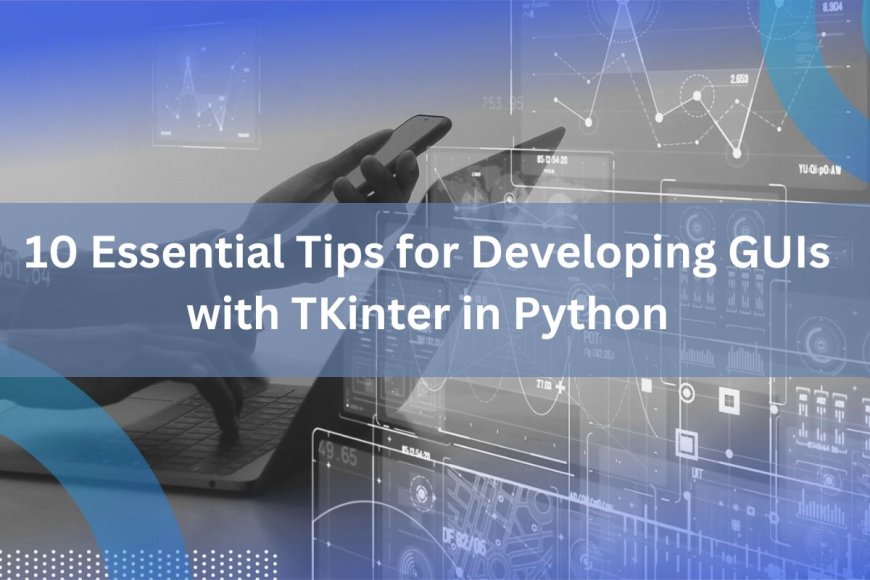
Introduction
Creating graphical user interfaces (GUIs) with Tkinter in Python can significantly enhance your application's user experience. To help you develop efficient and user-friendly interfaces, we’ve compiled ten essential tips. These guidelines cover layout planning, event handling, error management, and more, providing a solid foundation for your Tkinter projects.
What is Tkinter?
Tkinter is the standard graphical user interface (GUI) library for Python, included with most Python installations. It provides a powerful and flexible way to create desktop applications with a variety of widgets like buttons, labels, and text fields. Tkinter's simplicity and ease of use make it a popular choice for both beginners and experienced developers looking to build cross-platform GUI applications.
Tips for Developing GUIs with TKinter in Python
1. Understand the Basics of Tkinter
Tkinter is the standard GUI library for Python, and it’s included with most Python installations. Before diving into development, familiarize yourself with the basic components of Tkinter, such as windows, frames, widgets (like buttons and labels), and the layout managers (pack, grid, and place). Understanding these fundamental elements will make it easier to build complex interfaces.
2. Plan Your GUI Layout
Before coding, plan the layout of your GUI. Decide which widgets you need and where they should be placed. This step can save you time and help you visualize the final product. Sketching the layout on paper or using design software can provide a clear blueprint to follow during development.
3. Use Frames for Better Organization
Frames are containers that can hold other widgets. Using frames helps organize your GUI into logical sections, making it easier to manage and modify. For example, you can have separate frames for the header, body, and footer of your application. This approach keeps your code clean and your layout organized.
4. Master the Layout Managers
Tkinter provides three layout managers: pack, grid, and place. Each has its strengths and use cases.
-
Pack is straightforward and suitable for simple layouts where widgets are stacked vertically or horizontally.
-
Grid is more flexible and allows you to create complex layouts with rows and columns.
-
Place gives you precise control over widget positioning using x and y coordinates. Choose the right layout manager based on your needs and combine them when necessary.
5. Customize Widget Appearance
Tkinter widgets come with default styles, but customizing their appearance can enhance the user experience. You can modify properties like font, color, and size to match the look and feel of your application. Use the config method to update widget attributes and the ttk module for themed widgets that provide a more modern look.
6. Handle Events Properly
Event handling is crucial in GUI applications. Tkinter uses an event-driven programming model where actions (like button clicks) trigger events. Use the bind method to associate events with callback functions. Understand common events such as <Button-1> for mouse clicks and <Key> for keyboard presses to create responsive applications.
7. Implement Error Handling
Robust applications handle errors gracefully. Use try-except blocks to catch and manage exceptions, ensuring that your GUI remains responsive even when something goes wrong. Display user-friendly error messages using message boxes (from the messagebox module) to inform users of issues without crashing the application.
8. Keep the UI Responsive
A common issue in GUI development is the application becoming unresponsive during long-running tasks. To prevent this, offload time-consuming operations to separate threads or use asynchronous programming. The threading module allows you to run tasks in the background, while Tkinter’s after method can schedule functions to run after a delay, keeping the UI responsive.
9. Modularize Your Code
As your GUI application grows, keeping your code organized is vital. Break down your code into modules and classes. Define separate functions for creating different parts of the GUI, handling events, and performing specific tasks. This modular approach makes your code more readable, maintainable, and easier to debug.
10. Test Your Application Thoroughly
Thorough testing is essential to ensure your GUI works as expected. Test all user interactions, edge cases, and error scenarios. Regularly test your application on different operating systems if you plan to distribute it widely, as Tkinter behavior might vary slightly between platforms. Automated tests can also be beneficial for large projects.
Conclusion
Developing GUIs with Tkinter in Python can be an enjoyable and rewarding experience. By understanding the basics, planning your layout, using frames and layout managers, customizing widget appearance, handling events, implementing error handling, keeping the UI responsive, modularizing your code, and thoroughly testing your application, you can create effective and user-friendly interfaces. Remember that practice is key, so keep experimenting with different designs and functionalities to improve your skills. For those looking to enhance their knowledge further, consider joining a Python training institute in Surat, Nashik, Gurgaon, Kota, or other cities near you.
FAQs on 10 Essential Tips for Developing GUIs with Tkinter in Python
Q1: What is Tkinter?
A: Tkinter is the standard graphical user interface (GUI) library for Python. It allows developers to create desktop applications with various widgets like buttons, labels, and text fields.
Q2: Why should I use frames in Tkinter?
A: Frames help organize your GUI into logical sections, making it easier to manage, modify, and maintain the layout. They act as containers for other widgets, enhancing the structure of your application.
Q3: What are the three layout managers in Tkinter?
A: Tkinter provides three layout managers: pack, grid, and place. Pack is used for simple stacking of widgets, grid allows for complex layouts with rows and columns, and place gives precise control over widget positioning using coordinates.
Q4: How can I customize the appearance of Tkinter widgets?
A: You can customize widget appearance by modifying properties such as font, color, and size using the config method. Additionally, the ttk module offers themed widgets that provide a more modern look.
What's Your Reaction?

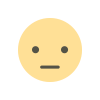
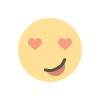



